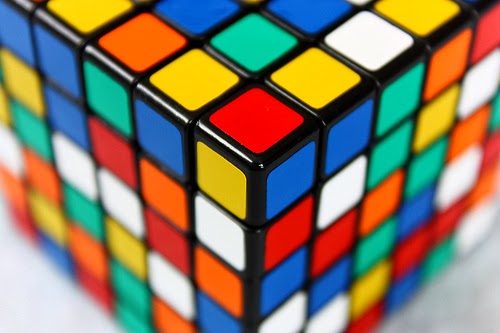
I split these examples into two specific categories which are good and dumb. Well, does this blog post dumb things? No, but still, this post is to show that there are such ways. Let me show them so that you could better grasp what fits the best.
class dr1
{
public static void main(String args[]) throws Exception
{
Class.forName("oracle.jdbc.driver.OracleDriver");
}
}
class dr2
{
public static void main(String args[]) throws Exception
{
// Choosing the driver at runtime
Class.forName(args[0]);
}
}
class dr3
{
public static void main(String args[]) throws Exception
{
// Choosing the driver at runtime by a temporary
// property
Class.forName(System.getProperty("my.driver"));
}
}
For executing this, you should write
java -Dmy.driver=oracle.jdbc.driver.OracleDriver dr3
The -D option is followed by the property name (without space) and = followed by the driver class. Don't forget the class name. It is damn important!
Note: The second, third and fourth ways have a common problem. Here if we aren't decisive about which driver to use and give it at runtime we need to change the URL (to connect to the database) too. Because, different drivers have different urls. So, there must be some conditional logic written which connects to the database via appropriate URL corresponding to the given class name. This becomes a bit messy. Isn't it?
class dr4
{
public static void main(String args[])
{
// Choosing the driver at runtime by a permanent
// property
System.out.println("Driver loaded "+System.getProperty("jdbc.drivers"));
}
}
Well, where is the code? I'll explain, everything lies in executing the program. You should execute this in this way.
java -Djdbc.drivers=oracle.jdbc.driver.OracleDriver dr4
Now, this loads the driver. The jdbc.drivers is an environment property of Java that corresponds to the JDBC drivers. The default value for this property is null. While executing, you are changing its value to the driver class name.
3 Dumb ways
class dr5
{
public static void main(String args[])
{
// Creating an object for the driver class
new oracle.jdbc.driver.OracleDriver();
}
}
I call this dumb because, we are creating an object for this class which is absolutely unnecessary. Here is a simple question to you
Why create an object for a class when you are not going to use it? If you want to load the static block of the class? Isn't it enough to load the class into the memory using Class.forName() instead of creating an object for it?
class dr6
{
public static void main(String args[]) throws Exception
{
// Using the registerDriver
DriverManager.registerDriver(new oracle.jdbc.driver.OracleDriver());
}
}
This way is double-dumb because you are loading the driver twice. Yes, the static block of the oracle.jdbc.driver.OracleDriver already contains a call to the registerDriver(). The statement, new oracle.jdbc.driver.OracleDriver(); loads it into memory (therefore, static block is executed) and creates an object for the driver class and then register the driver.
class dr7 extends oracle.jdbc.driver.OracleDriver
{
public static void main(String args[])
{
System.out.println("Driver is loaded.");
}
}
This is triple-dumb because Java doesn't support multiple inheritance. What if we want to extend this class with some other class?
This method however, does the job. The static block of the super class (the driver class here) gets executed and the regsiterDriver() is called. Here is a question to you.
Why extend a class when you can simply create an object for it? Also, don't forget to answer the previously posed one, which is the tail of this question.
Photo Credit: S3V3R!7Y
No comments:
Post a Comment