An example on keeping JDialog on the center of the screen and on the center of JFrame in Java.
Example
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
class CenterJDialog extends JFrame
{
JButton jb;
JDialog jd;
JFrame thisFrame;
public CenterJDialog()
{
// Set frame properties
setTitle("Center a JDialog");
setSize(400,400);
setLayout(new FlowLayout());
setDefaultCloseOperation(EXIT_ON_CLOSE);
setLocationRelativeTo(null);
setVisible(true);
// Set value of thisFrame to current object
thisFrame=this;
// Create a JButton
jb=new JButton("Click me to open JDialog :)");
// Add JButton to the JFrame
add(jb);
// Add action listener for jb
jb.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent ae)
{
// Set dialog properties
jd=new JDialog(thisFrame);
jd.setTitle("I am a JDialog");
jd.setLayout(new FlowLayout());
// Add sample components
jd.add(new JTextField(20));
jd.add(new JButton("Button"));
jd.setVisible(true);
jd.pack();
// Set location centered for JFrame
jd.setLocationRelativeTo(thisFrame);
// Set location centered for screen
//jd.setLocationRelativeTo(null);
}
});
}
public static void main(String args[])
{
new CenterJDialog();
}
}
Output
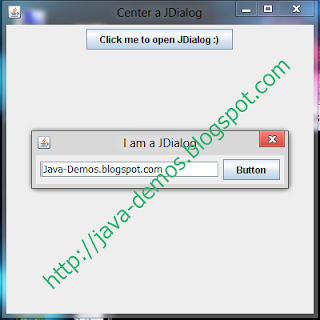 |
Center JDialog for JFrame [here] for screen [src] |
No comments:
Post a Comment