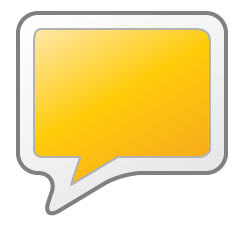
What's here?
In this post, i will be drawing a table of 3 columns and 5 rows on a Swing tooltip along with a <h2> heading with a white background. When it comes to the program, a short logic is written to draw the table on swing tooltip. While this logic is not needed, it is written instead for the sake of brevity. We will be using no classes outside the Swing and will be using no event handling in the program.Shall we draw? Yes!
import javax.swing.*;
import java.awt.*;
class TableTooltip extends JFrame
{
private JButton b;
public TableTooltip()
{
createAndShowGUI();
}
private void createAndShowGUI()
{
setTitle("Table on a Tooltip");
setLayout(new FlowLayout());
setDefaultCloseOperation(EXIT_ON_CLOSE);
setSize(400,400);
setVisible(true);
b=new JButton("Button");
add(b);
String text="<html><body bgcolor=white><h2 align=center>This is a Table</h2><br/><table border=1>";
for(int i=0;i<5;i++)
{
text+="<tr>";
for(int j=0;j<3;j++)
{
text+="<td>[Row "+i+", Column "+j+"]</td>";
}
text+="</tr>";
}
text+="</table></body></html>";
b.setToolTipText(text);
}
public static void main(String args[])
{
new TableTooltip();
}
}
Output of the TableTooltip program
Drawing table on Swing tooltip |
Explaining the table drawing
Here i have used HTML with Swing in order to draw the table on the tooltip. HTML contains a tag called the <table> which is used to draw a table and border attribute sets the border thickness which is 1 here.
<body> tag represents the body of the page.
bgcolor is an attribute which points to the background of body. white is the value for the bgcolor attribute.
<h2> tag represents the second heading which is smaller than the 1st and bigger than 3,4,5 and 6.align is an attribute which defines the alignment of the text. center is the value for the attribute align.
<tr> represents a table row and <td> represents a table data. A <tr> usually contains <td>.
for(int i=0;i<5;i++) Loop till 5 times starting from 0 as i value. This outer loop is written for table row.
text+="<tr>"; This creates a new table row each time the outer loop executes.
for(int j=0;j<3;j++) Loop till 3 times starting from 0 as j value. This inner loop is written for table data (a table cell).
text+="<td>[Row "+i+", Column "+j+"]</td>"; Open the <td> tag and write into the cell. The row and column number.
text+="</tr>"; Close the table row once after the inner loop has finished.
b.setToolTipText(text); Put the tool tip text for the JButton.
No comments:
Post a Comment